
Admin
January 14, 2024
Learn React, Vite, TypeScript, and Tailwind CSS project setup in 15 minutes 😎:
1. Create a Vite Project:
- Open your terminal and run:
npm create vite@latest my-react-app --template react-ts
- Navigate into the project directory:
cd my-react-app
2. Install Tailwind CSS:
- Install the required dependencies:
npm install -D tailwindcss postcss autoprefixer
- Create Tailwind's configuration files:
npx tailwindcss init -p
3. Configure Source Paths:
- In tailwind.config.cjs, add paths to your template files:
module.exports = {
content: [
"./index.html",
"./src/**/*.{js,ts,jsx,tsx}",
],
theme: {
extend: {},
},
plugins: [],
};
4. Add Tailwind Directives:
- In src/index.css, add the Tailwind directives:
@tailwind base;
@tailwind components;
@tailwind utilities;
4. Create the postcss.config.cjs file:
- Next step is to create a file named postcss.config.cjs in the root directory of your project.
5. Add PostCSS configuration:
- Paste the following code into the postcss.config.cjs file
module.exports = {
plugins: {
tailwindcss: {},
autoprefixer: {},
},
};
6. No further actions needed:
- Vite automatically picks up this configuration without any additional setup.
Key points:
- The postcss.config.cjs file is crucial for managing PostCSS plugins, including Tailwind CSS and Autoprefixer.
- Vite's built-in PostCSS support streamlines the process, eliminating manual configuration within vite.config.js.
With this addition, you'll have a complete setup for React, Vite, TypeScript, and Tailwind CSS, ready for your development work.
7. Create a React Component:
- Create a basic component in src/App.tsx:
import React from 'react';
const App = () => {
return (
<div className="bg-gray-200 h-screen flex items-center justify-center">
<h1 className="text-3xl font-bold text-blue-500">Hello, Vite + Tailwind!</h1>
</div>
);
};
export default App;
8. Run the Development Server:
- Start the development server:
npm run dev
- Open http://localhost:5173 in your browser to see your app.
Additional Tips:
- Customize Tailwind: Explore Tailwind's configuration options in tailwind.config.cjs to tailor it to your project's needs.
- Tailwind IntelliSense: Install the Tailwind CSS IntelliSense extension for your code editor to get helpful class suggestions.
- Hot Reloading: Vite's hot module replacement ensures instant updates as you make changes to your code and styles.
Congratulations! You've successfully set up React, Vite, TypeScript, and Tailwind CSS. Now you can enjoy a fast and efficient development workflow with a powerful styling framework.
Recent Post
“Unlock Next-Level Web Development: Dive into Next.js 15’s Exciting Features!” +1
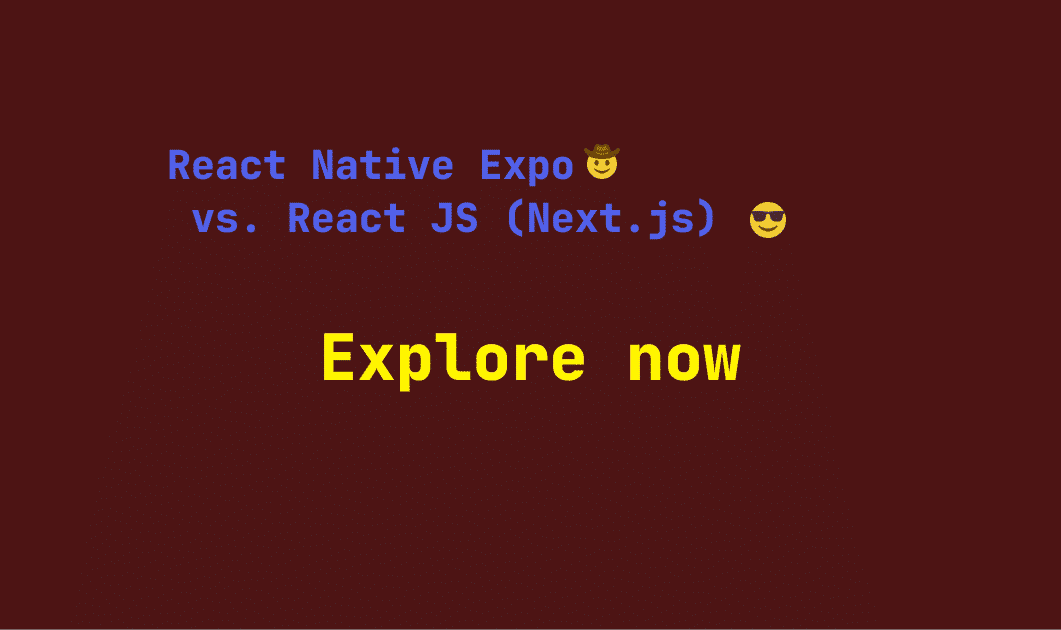
React Native Expo vs. React JS (Next.js): A Comprehensive Guide for Choosing the Right
.png&w=3840&q=75)
Next.js: React's Next Evolution
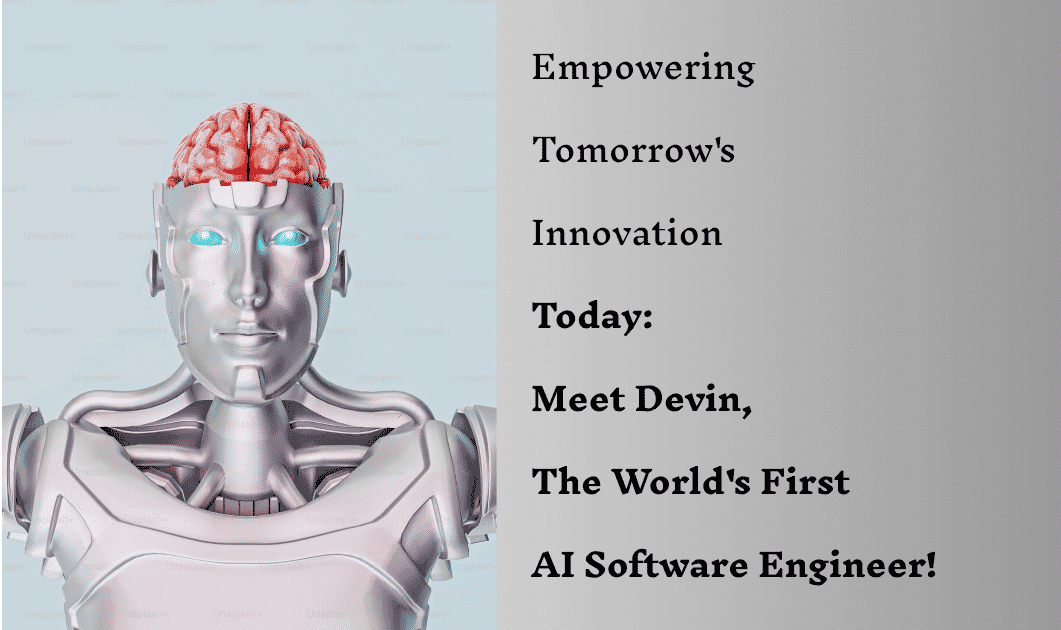
Meet Devin , The World's First Software Engineer!
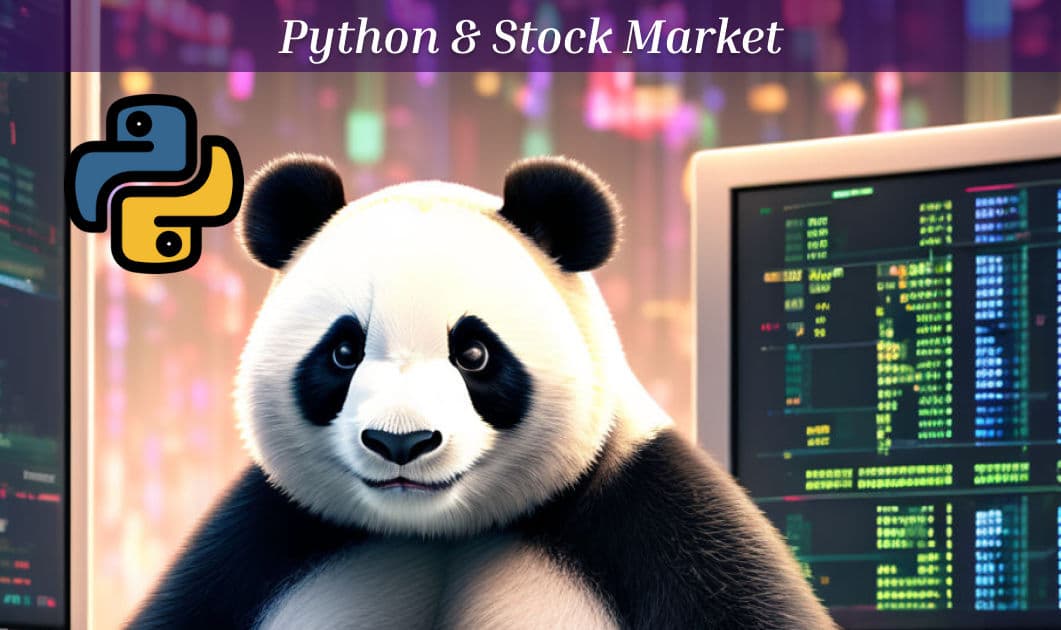
Python and Stock Market: A Simple and Brief Overview
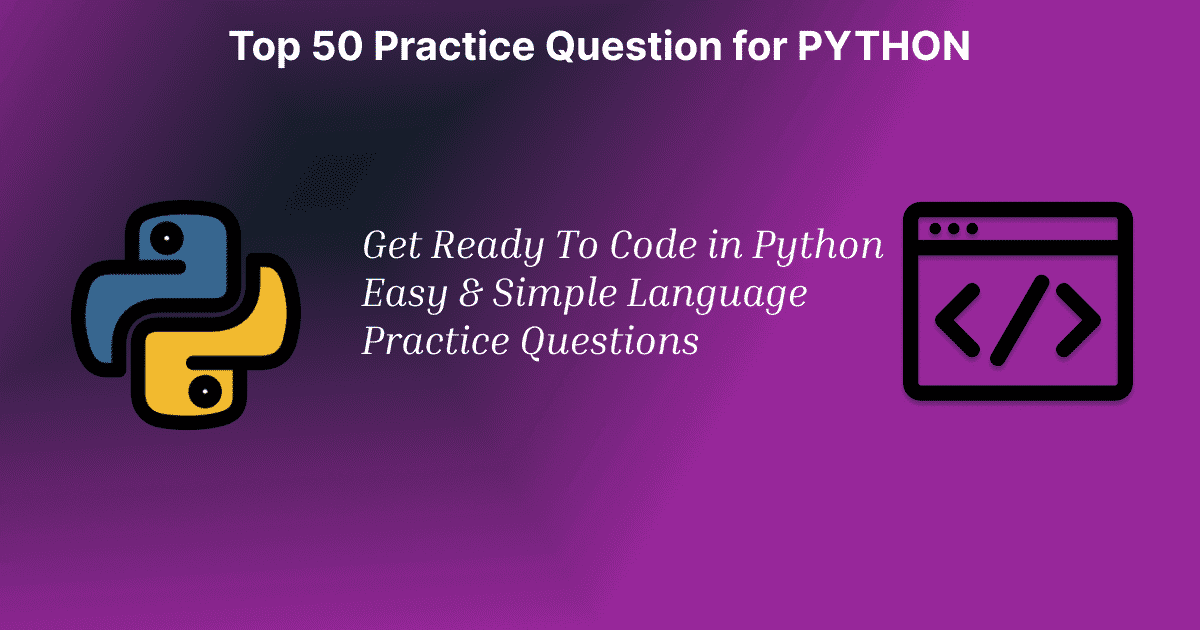
Python Mastery Quest: 50 Engaging Challenges to Level Up Your Coding Skills
.png&w=3840&q=75)
A Guide to ESLint: Enhancing JavaScript Code Quality
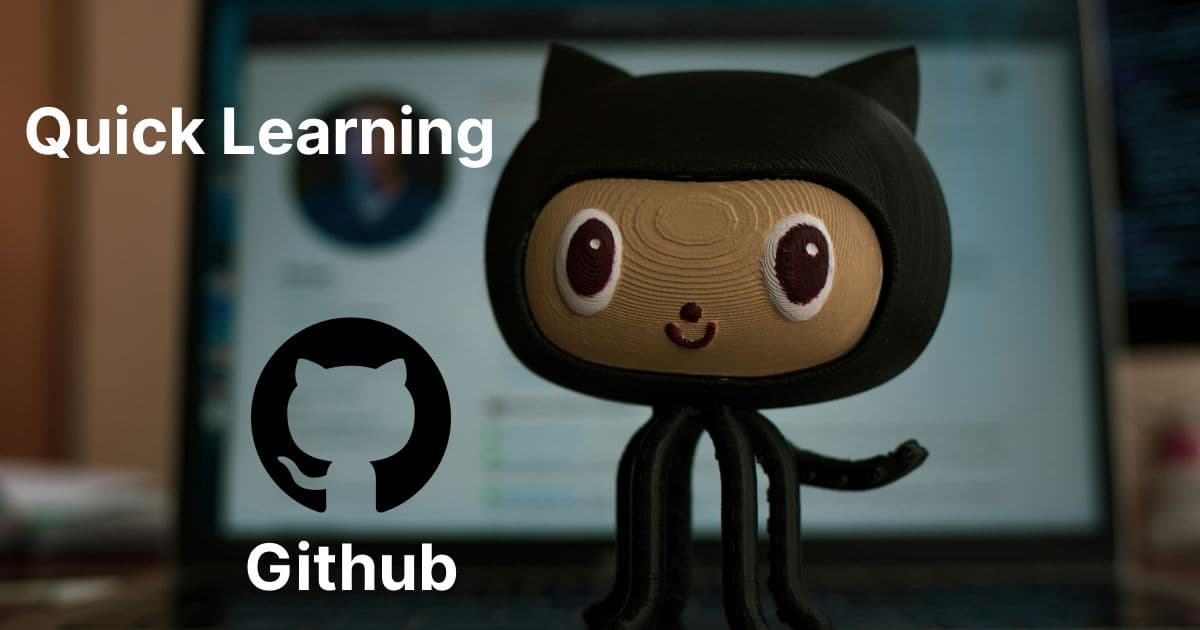
GitHub for Beginners: A Step-by-Step Guide to Pushing Your Code - Mastering the Basics and Essential Commands
.png&w=3840&q=75)
Python Beginning: Simple Calculator
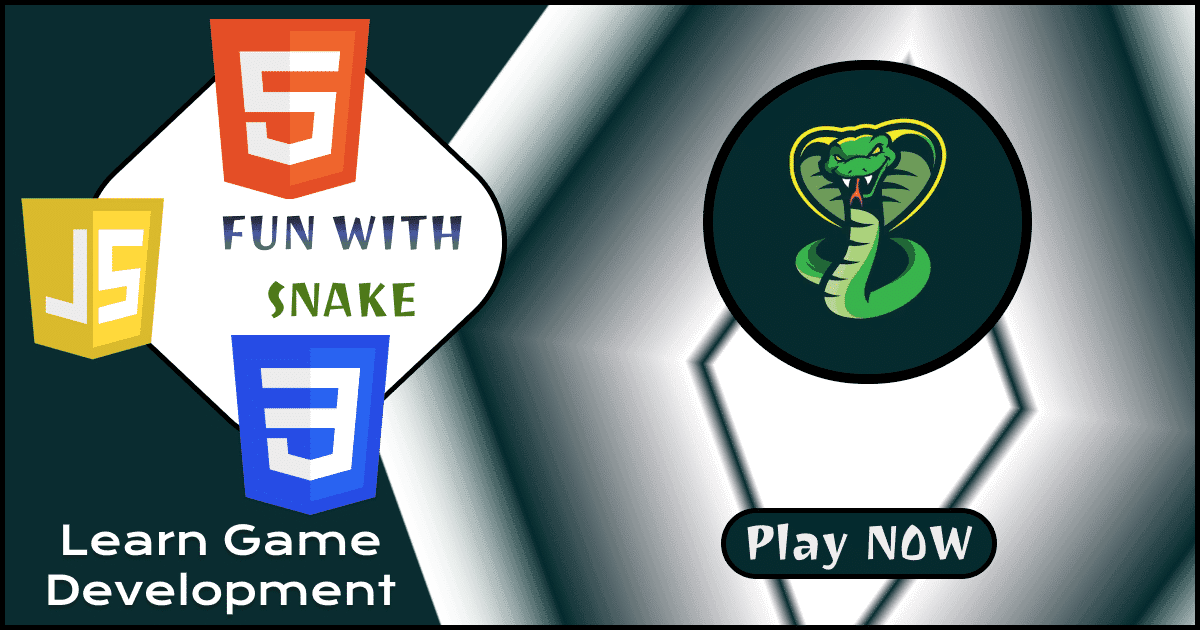
Snake Game Code: A Beginner's Guide
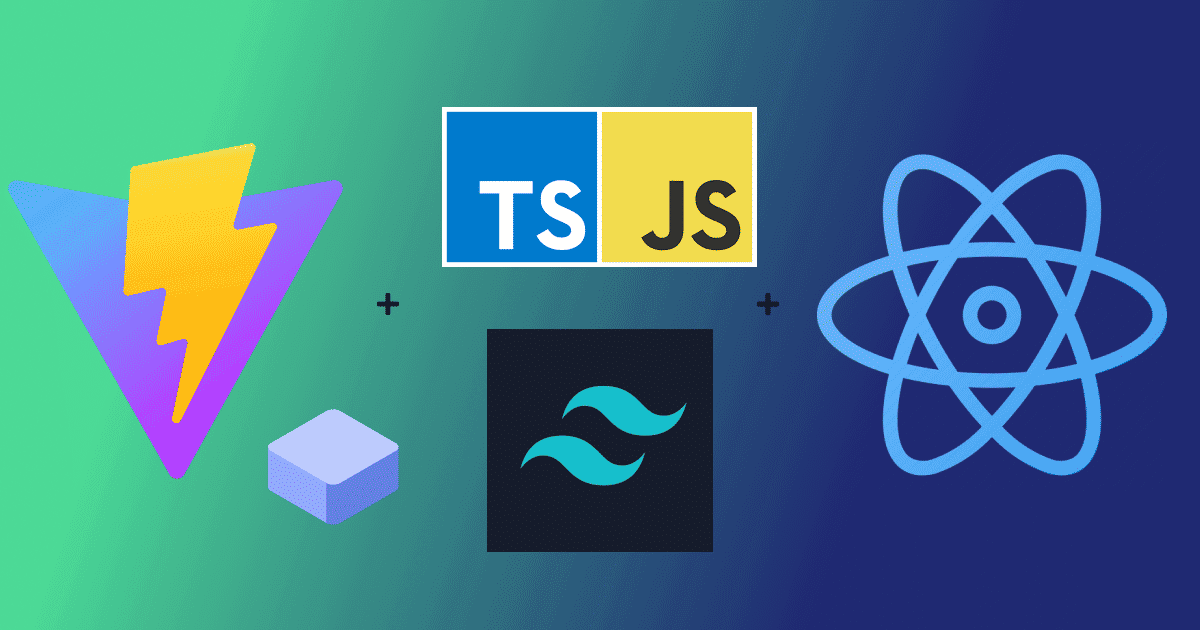
Easy Setup: Vite, React, TypeScript, and Tailwind Project Guide
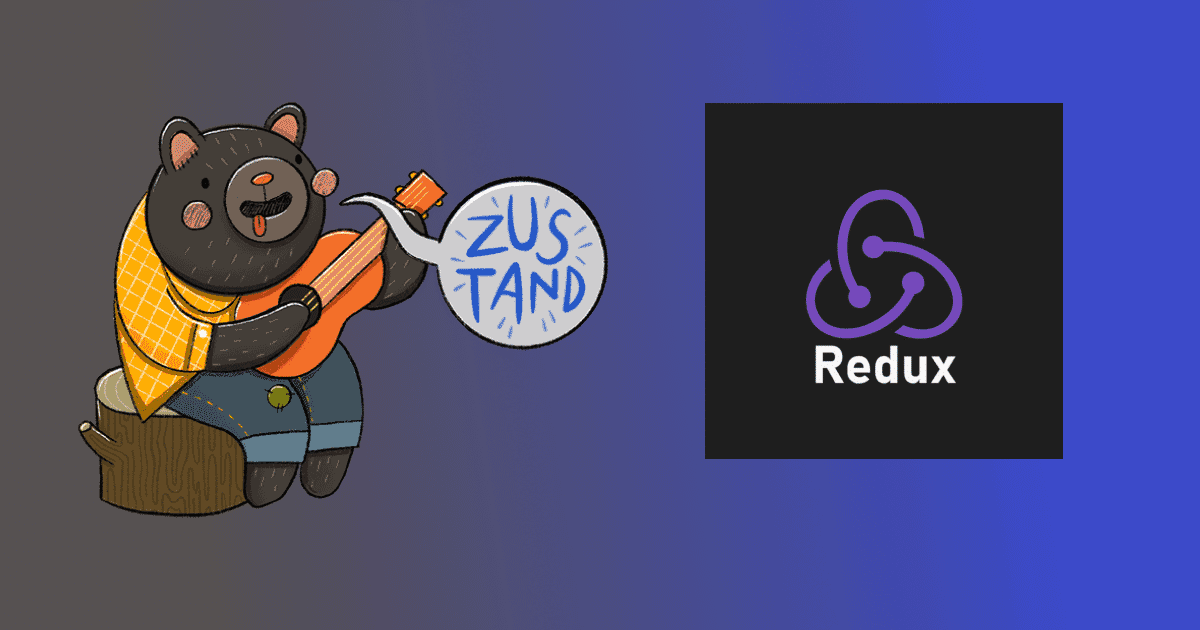
Why you should prefer Zustand over Redux Toolkit?
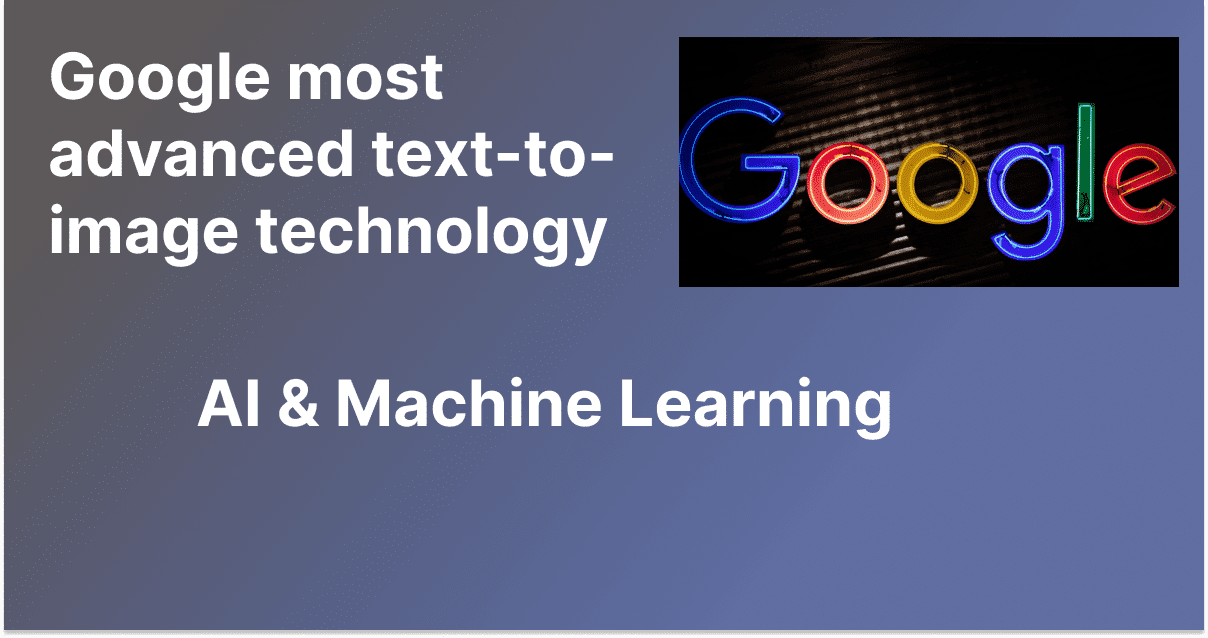
Google launches Imagen 2, better than DALL.E 2?
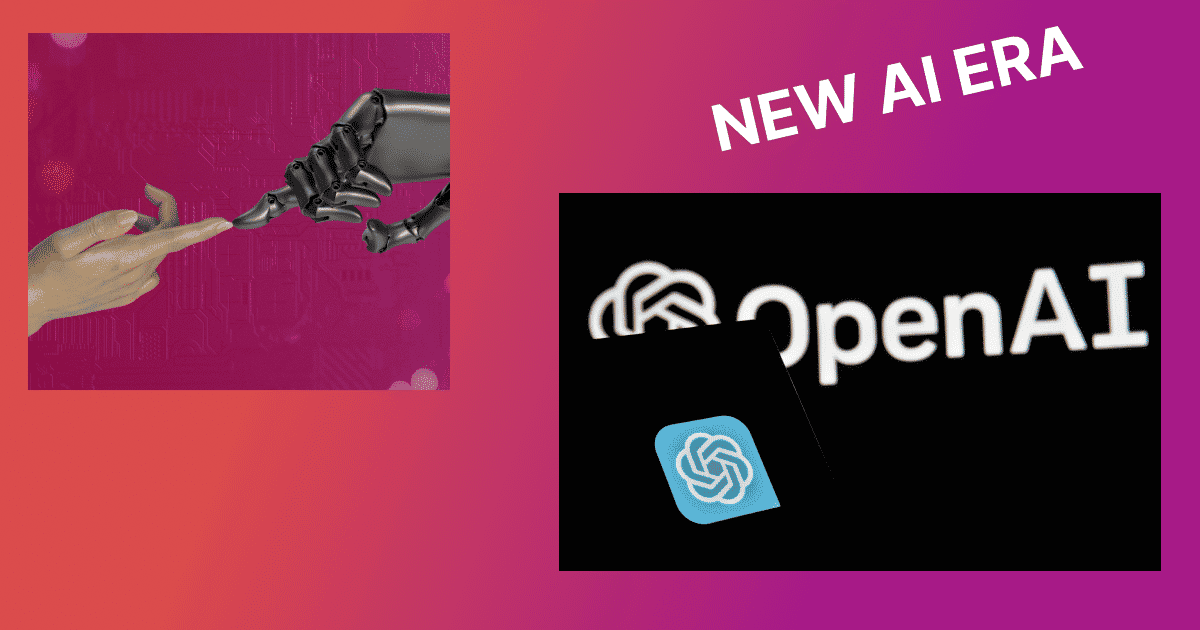
Decoding the New AI Era: Is Gemini by Google the Ultimate ChatGPT Alternative?